skunkmere
Member
Ive been working on this with no coding experience. finally got it to work. here's my work for you all that want to make one.
The site i got the instructions from : http://fishtankprojects.com/diy-aquarium-projects/arduino-controlled-dosing-pumps.html#comment-309
the code on the site has errors and i cleaned it up and changed it to work a bit different. here is the code:
#include <Wire.h>
#define DS1307_I2C_ADDRESS 0x68
// Convert normal decimal numbers to binary coded decimal
byte decToBcd(byte val)
{
return ( (val/10*16) + (val%10) );
}
// Convert binary coded decimal to normal decimal numbers
byte bcdToDec(byte val)
{
return ( (val/16*10) + (val%16) );
}
// 1) Sets the date and time on the ds1307
// 2) Starts the clock
// 3) Sets hour mode to 24 hour clock
// Assumes you're passing in valid numbers
void setDateDs1307(
byte second, // 0-59
byte minute, // 0-59
byte hour, // 1-23
byte dayOfWeek, // 1-7
byte dayOfMonth, // 1-28/29/30/31
byte month, // 1-12
byte year) // 0-99
{
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.write(decToBcd(second)); // 0 to bit 7 starts the clock
Wire.write(decToBcd(minute));
Wire.write(decToBcd(hour)); // If you want 12 hour am/pm you need to set
// bit 6 (also need to change readDateDs1307)
Wire.write(decToBcd(dayOfWeek));
Wire.write(decToBcd(dayOfMonth));
Wire.write(decToBcd(month));
Wire.write(decToBcd(year));
Wire.endTransmission();
}
// Gets the date and time from the ds1307
void getDateDs1307(
byte *second,
byte *minute,
byte *hour,
byte *dayOfWeek,
byte *dayOfMonth,
byte *month,
byte *year)
{
// Reset the register pointer
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.endTransmission();
Wire.requestFrom(DS1307_I2C_ADDRESS, 7);
// A few of these need masks because certain bits are control bits
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f); // Need to change this if 12 hour am/pm
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
//define pins
int ato = 9;
int vinegar = 10;
//int motorPin3 = 11;
void setup() // run once, when the sketch starts
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
pinMode(ato, OUTPUT);
pinMode(vinegar, OUTPUT);
//pinMode(motorPin3, OUTPUT);
Wire.begin();
Serial.begin(9600);
// Change these values to what you want to set your clock to.
// You only need to run this the first time you setup your RTC.
// Set the correct value below and un comment it to run it.
/* remove line to set time on RTC
second = 00;
minute = 40;
hour = 12;
dayOfWeek = 2;
dayOfMonth = 17;
month = 3;
year = 14;
setDateDs1307(second, minute, hour, dayOfWeek, dayOfMonth, month, year);
*/ //Remove Line to set time and Date
}
void loop() // run over and over again
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
// this prints the output to the serial window (tools > serial monitor in arduino) and is great for testing
getDateDs1307(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month, &year);
Serial.print(hour, DEC);
Serial.print(":");
Serial.print(minute, DEC);
Serial.print(":");
Serial.print(second, DEC);
Serial.println();
// Set the time you want the motors to kick in
if((hour == 10)&(minute == 00)&(second==00)){
Serial.print("Dosing");
Serial.println(":");
Serial.println("ATO");
analogWrite(ato, 255);
delay(300000); // =5min set how long you want the motor to run... 2000 = aprox 1ml 25ml/sec
analogWrite(ato, 0);}
if((hour == 10)&(minute == 15)&(second==00)){
Serial.print("Dosing");
Serial.println(":");
Serial.println("Vinegar");
analogWrite(vinegar, 255);
delay(12000); // =6sec set how long you want the motor to run... 2000 = aprox 1ml 27ml/sec
analogWrite(vinegar, 0);}
/*
if((hour == 10)&(minute == 05)&(second==00)){
delay(10000); //Time between pump running
Serial.println("Vine");
analogWrite(motorPin3, 255);
delay(60000); // set how long you want the motor to run... 1000 = aprox 1ml
analogWrite(motorPin3, 0);}
*/
}
Here are some pictures of my enclosure i made with acrylic and a laser cutter.
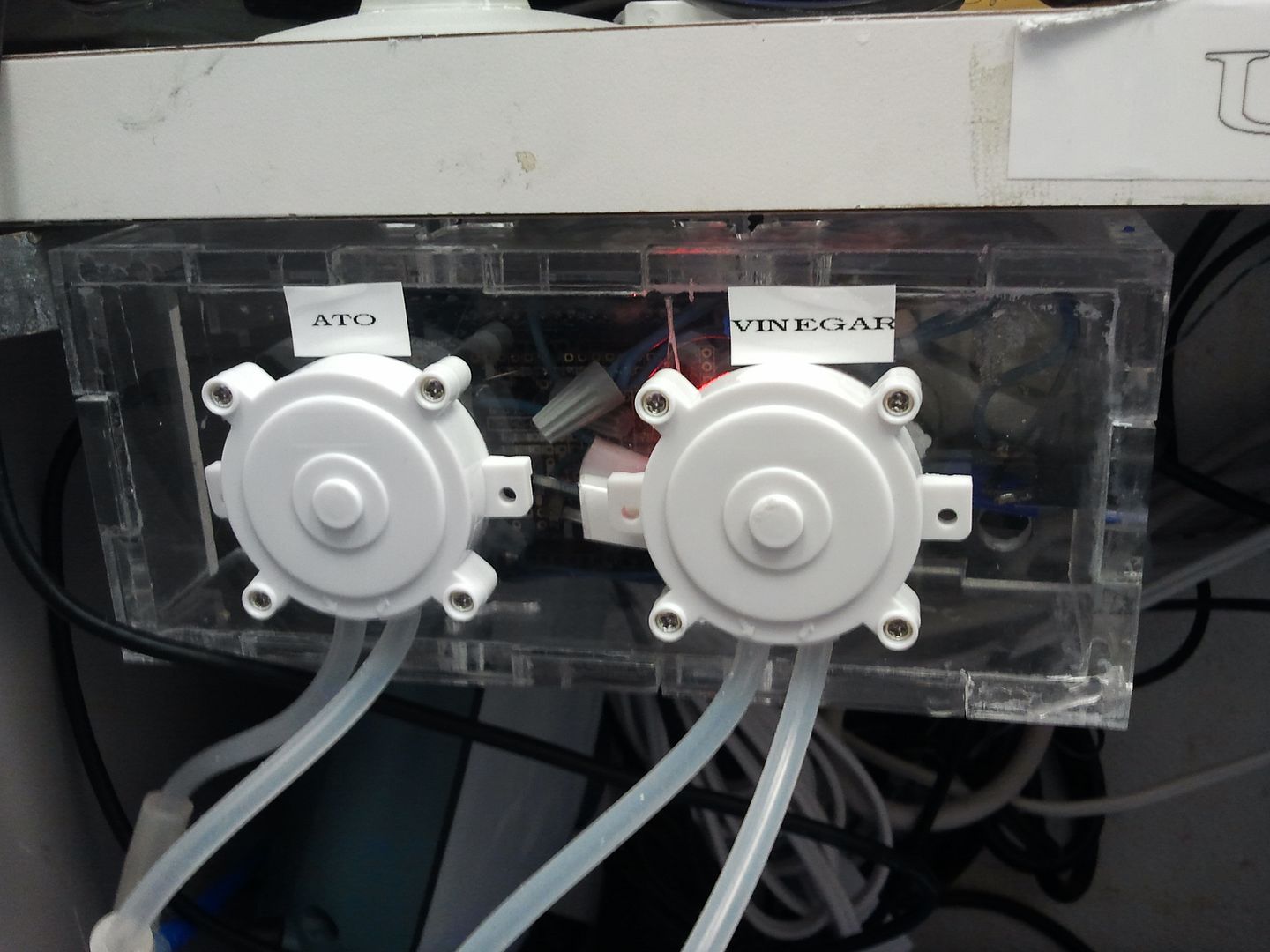
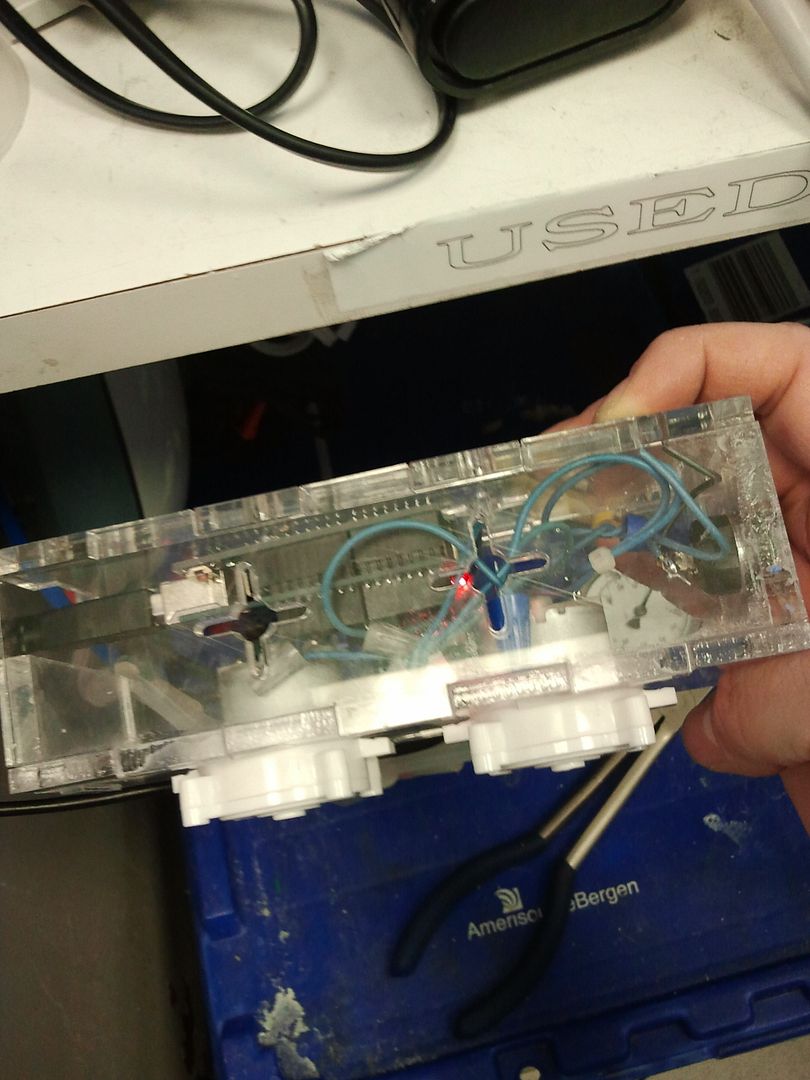
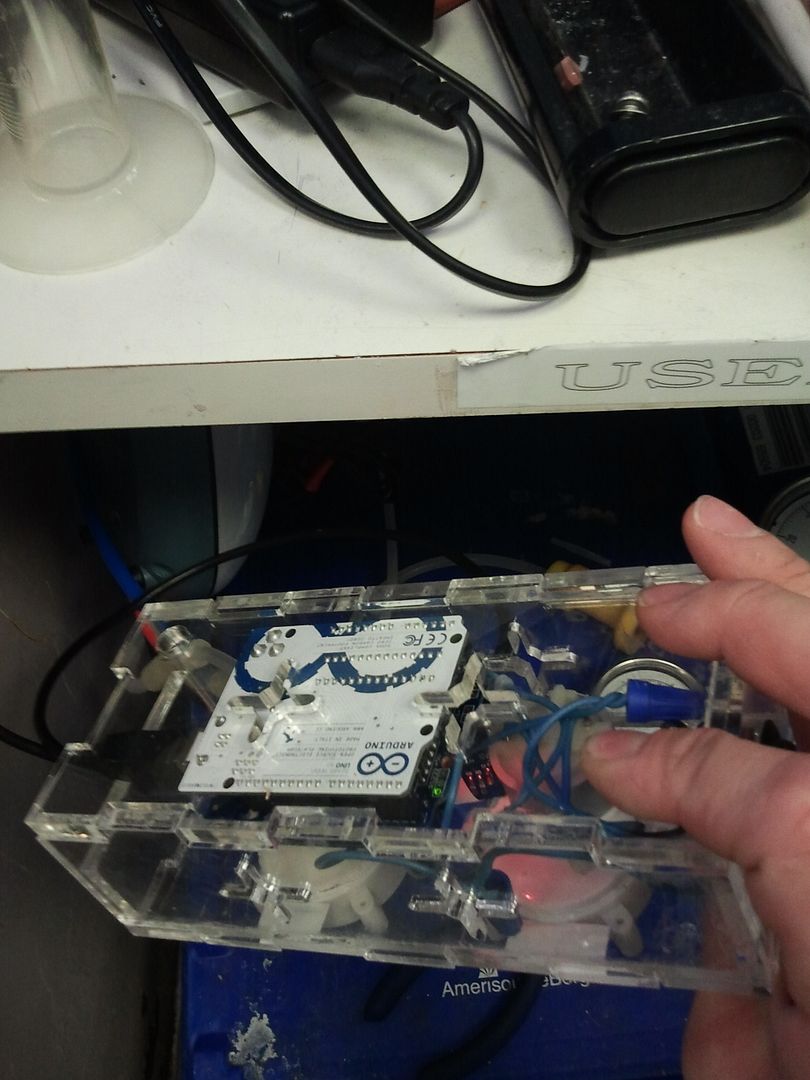
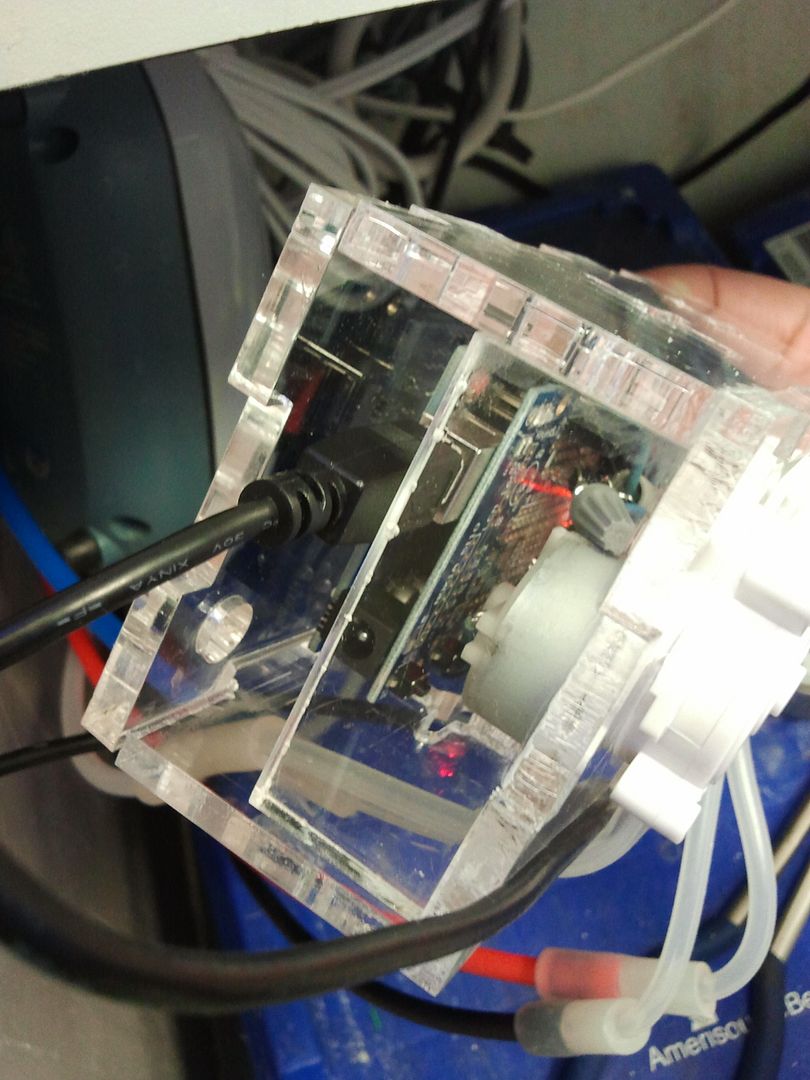
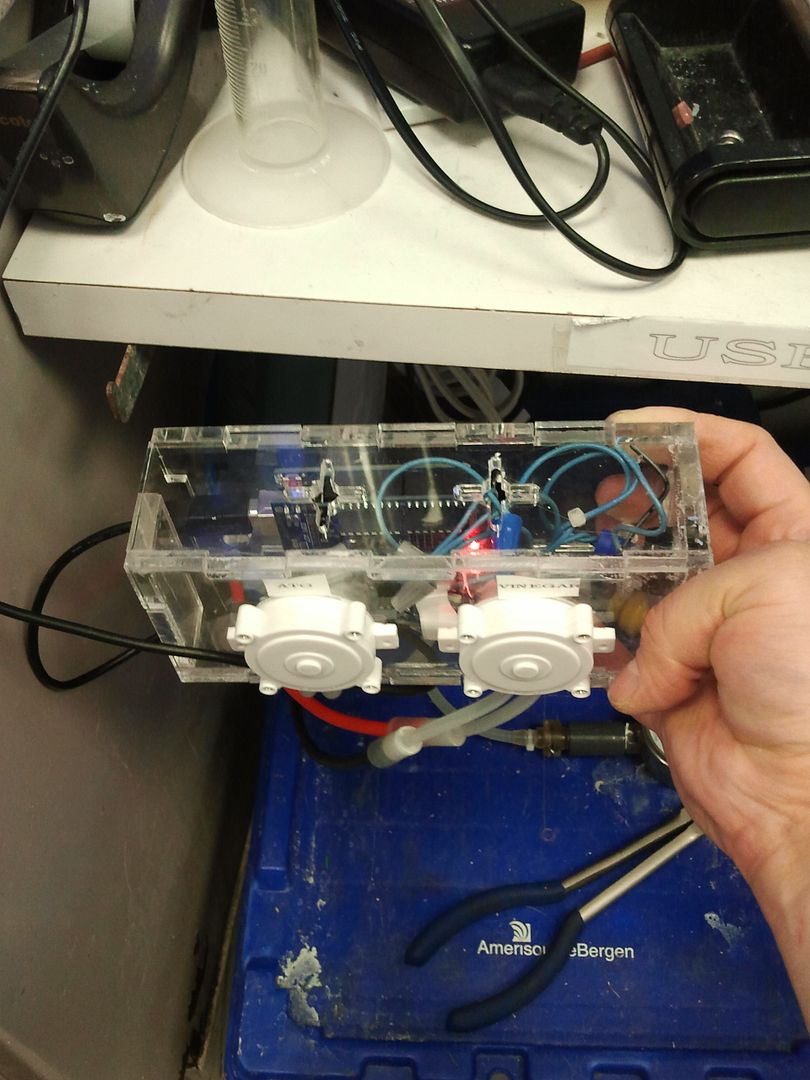
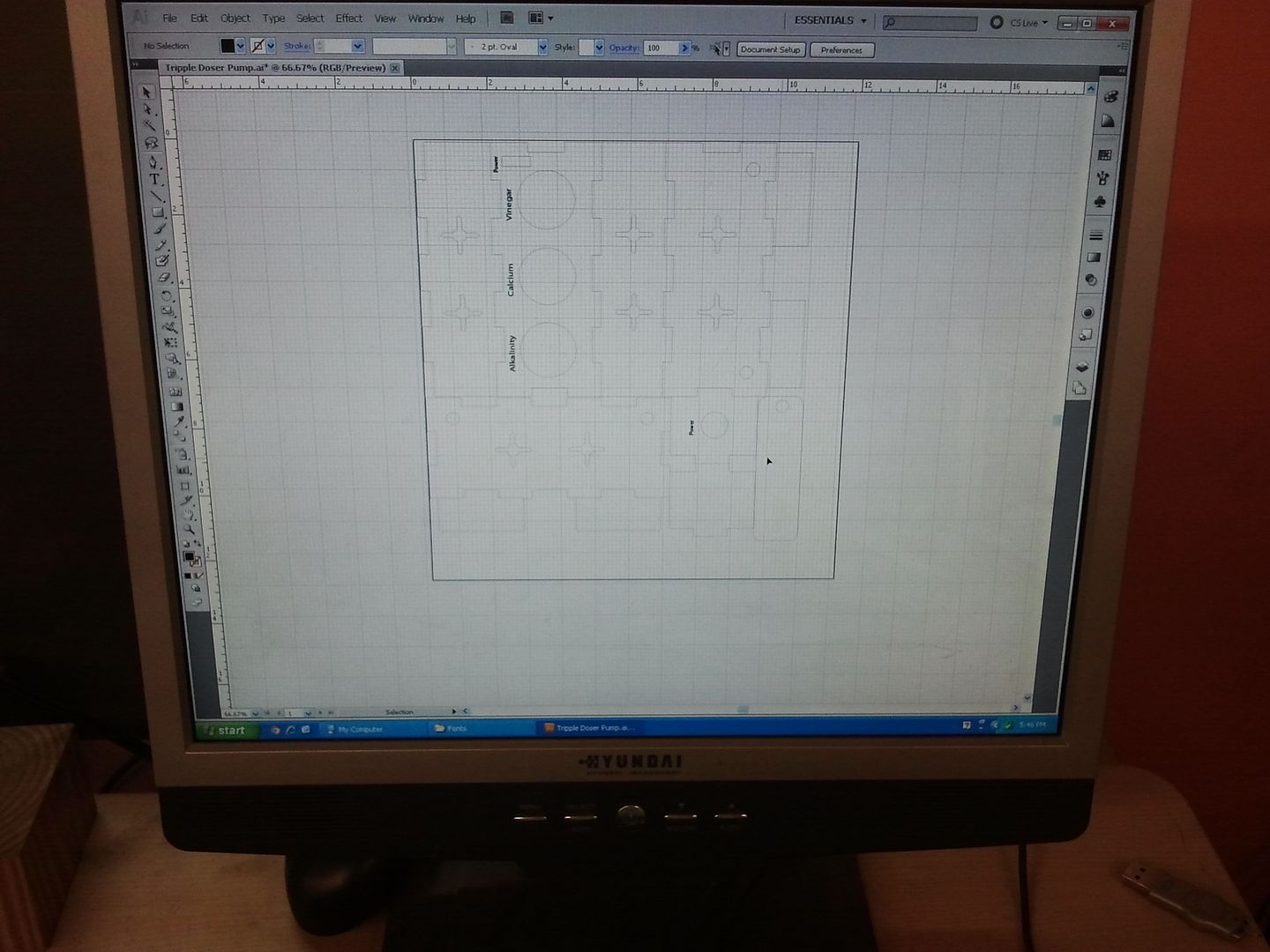
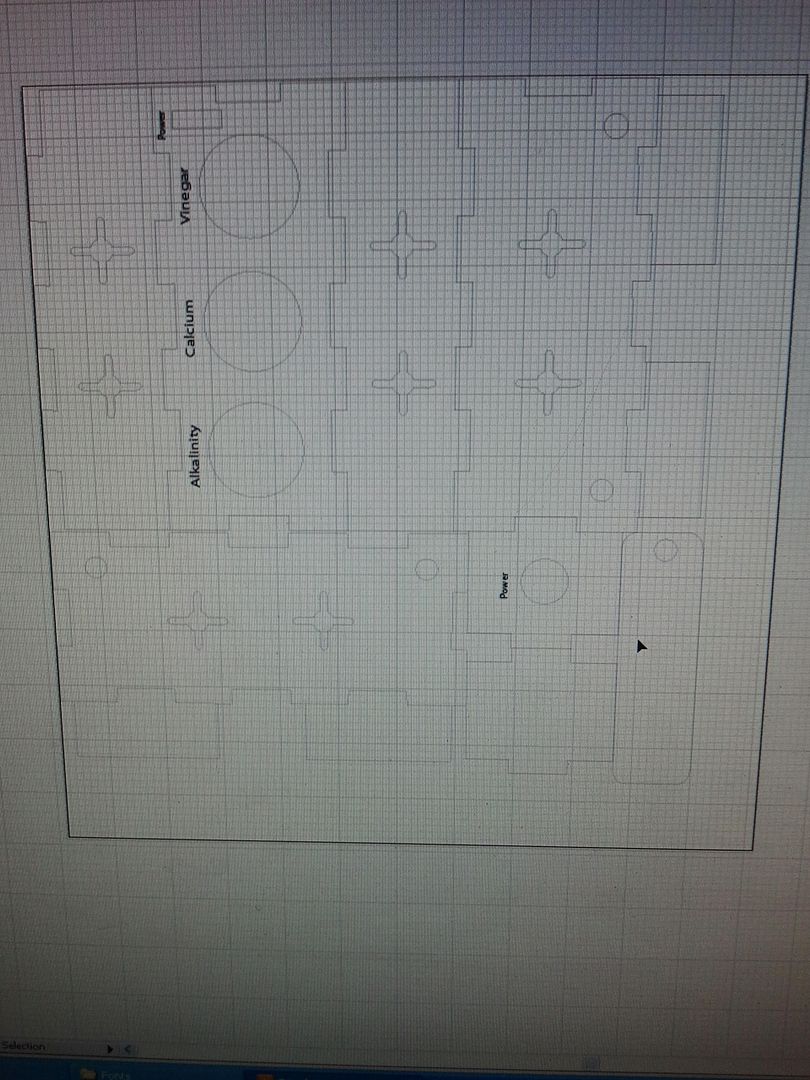

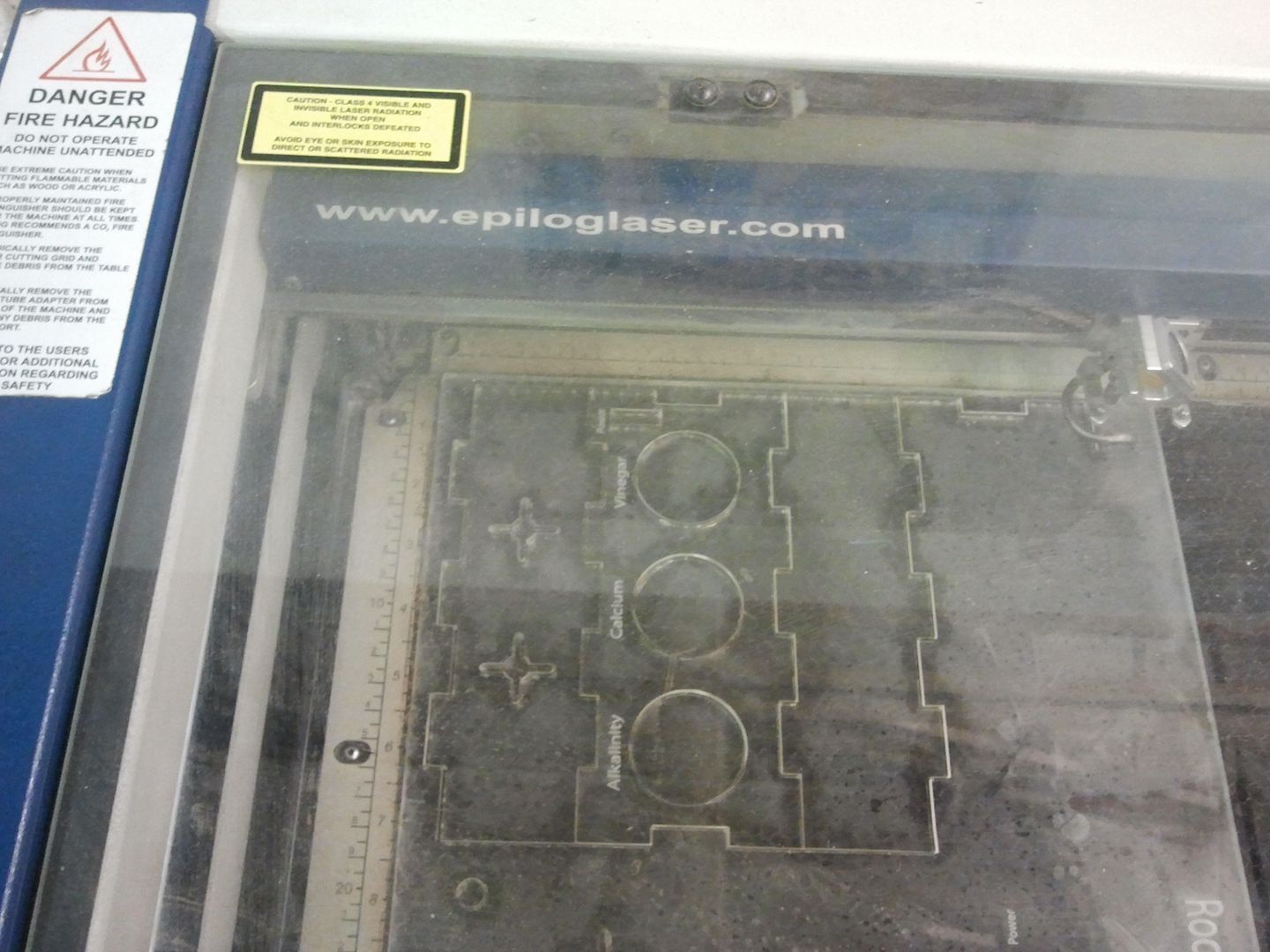
Files :
https://mega.co.nz/#!AZAHGBiT!FSgOTR1c_y0xpakP-_suoHZv09VBAQTTLZ390Gas-RY
I ordered these pumps:
http://www.ebay.com/itm/201020653246?ssPageName=STRK:MEWNX:IT&_trksid=p3984.m1497.l2649
25-30 ml a minute so its very slow dosing.
If anyone can improve on this please post suggestions and maybe we can make a better doser. Maybe with a LCD screen with buttons to change dosing and times. This code only allows you to program the dosing through the arduino coding program. The original site i got this from is not being updated, i tried to conact the owner for help on the code and i got no response. But thanks to him im learning how to use a Ardunio.
The site i got the instructions from : http://fishtankprojects.com/diy-aquarium-projects/arduino-controlled-dosing-pumps.html#comment-309
the code on the site has errors and i cleaned it up and changed it to work a bit different. here is the code:
#include <Wire.h>
#define DS1307_I2C_ADDRESS 0x68
// Convert normal decimal numbers to binary coded decimal
byte decToBcd(byte val)
{
return ( (val/10*16) + (val%10) );
}
// Convert binary coded decimal to normal decimal numbers
byte bcdToDec(byte val)
{
return ( (val/16*10) + (val%16) );
}
// 1) Sets the date and time on the ds1307
// 2) Starts the clock
// 3) Sets hour mode to 24 hour clock
// Assumes you're passing in valid numbers
void setDateDs1307(
byte second, // 0-59
byte minute, // 0-59
byte hour, // 1-23
byte dayOfWeek, // 1-7
byte dayOfMonth, // 1-28/29/30/31
byte month, // 1-12
byte year) // 0-99
{
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.write(decToBcd(second)); // 0 to bit 7 starts the clock
Wire.write(decToBcd(minute));
Wire.write(decToBcd(hour)); // If you want 12 hour am/pm you need to set
// bit 6 (also need to change readDateDs1307)
Wire.write(decToBcd(dayOfWeek));
Wire.write(decToBcd(dayOfMonth));
Wire.write(decToBcd(month));
Wire.write(decToBcd(year));
Wire.endTransmission();
}
// Gets the date and time from the ds1307
void getDateDs1307(
byte *second,
byte *minute,
byte *hour,
byte *dayOfWeek,
byte *dayOfMonth,
byte *month,
byte *year)
{
// Reset the register pointer
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.endTransmission();
Wire.requestFrom(DS1307_I2C_ADDRESS, 7);
// A few of these need masks because certain bits are control bits
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f); // Need to change this if 12 hour am/pm
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
//define pins
int ato = 9;
int vinegar = 10;
//int motorPin3 = 11;
void setup() // run once, when the sketch starts
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
pinMode(ato, OUTPUT);
pinMode(vinegar, OUTPUT);
//pinMode(motorPin3, OUTPUT);
Wire.begin();
Serial.begin(9600);
// Change these values to what you want to set your clock to.
// You only need to run this the first time you setup your RTC.
// Set the correct value below and un comment it to run it.
/* remove line to set time on RTC
second = 00;
minute = 40;
hour = 12;
dayOfWeek = 2;
dayOfMonth = 17;
month = 3;
year = 14;
setDateDs1307(second, minute, hour, dayOfWeek, dayOfMonth, month, year);
*/ //Remove Line to set time and Date
}
void loop() // run over and over again
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
// this prints the output to the serial window (tools > serial monitor in arduino) and is great for testing
getDateDs1307(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month, &year);
Serial.print(hour, DEC);
Serial.print(":");
Serial.print(minute, DEC);
Serial.print(":");
Serial.print(second, DEC);
Serial.println();
// Set the time you want the motors to kick in
if((hour == 10)&(minute == 00)&(second==00)){
Serial.print("Dosing");
Serial.println(":");
Serial.println("ATO");
analogWrite(ato, 255);
delay(300000); // =5min set how long you want the motor to run... 2000 = aprox 1ml 25ml/sec
analogWrite(ato, 0);}
if((hour == 10)&(minute == 15)&(second==00)){
Serial.print("Dosing");
Serial.println(":");
Serial.println("Vinegar");
analogWrite(vinegar, 255);
delay(12000); // =6sec set how long you want the motor to run... 2000 = aprox 1ml 27ml/sec
analogWrite(vinegar, 0);}
/*
if((hour == 10)&(minute == 05)&(second==00)){
delay(10000); //Time between pump running
Serial.println("Vine");
analogWrite(motorPin3, 255);
delay(60000); // set how long you want the motor to run... 1000 = aprox 1ml
analogWrite(motorPin3, 0);}
*/
}
Here are some pictures of my enclosure i made with acrylic and a laser cutter.
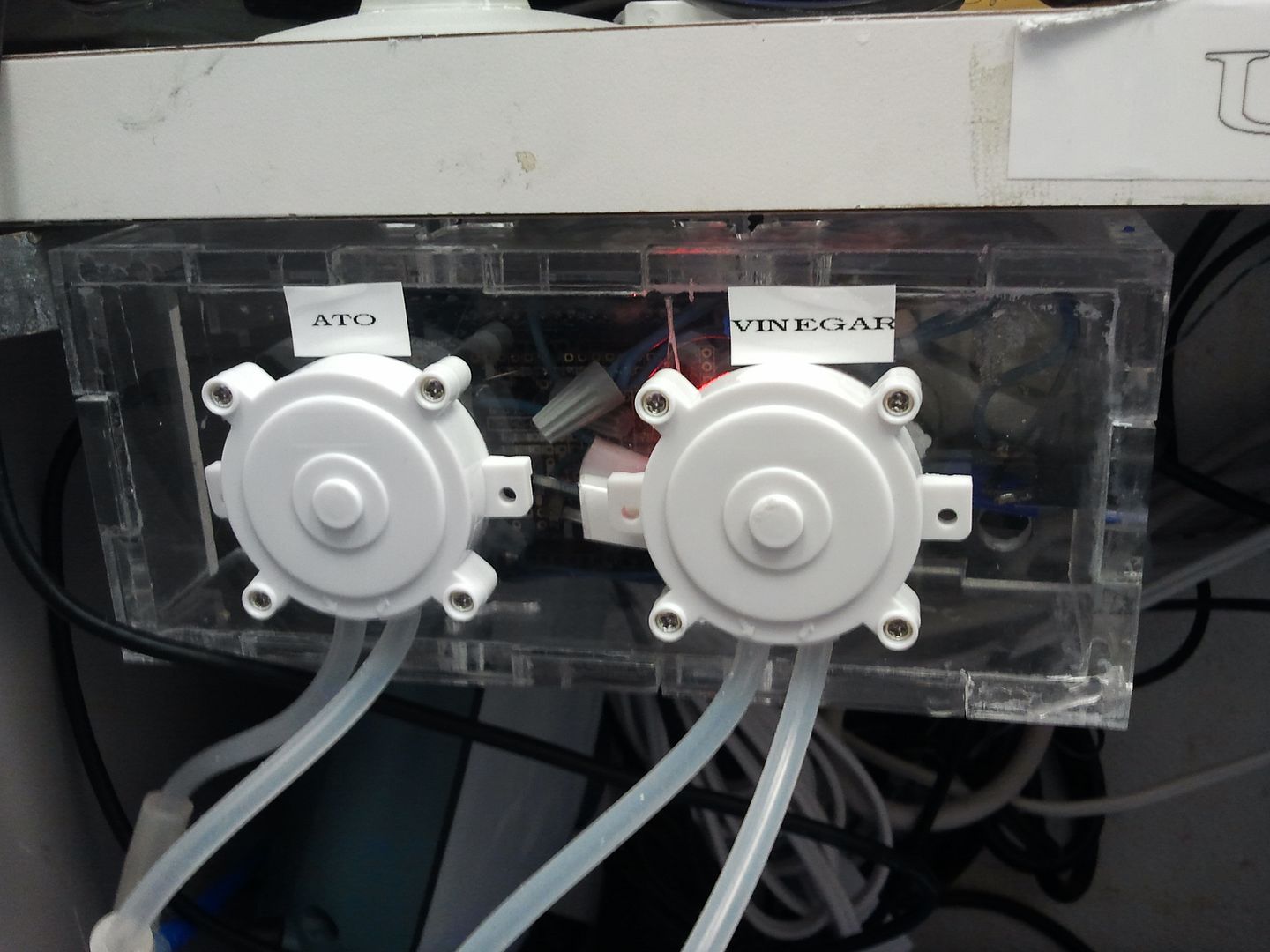
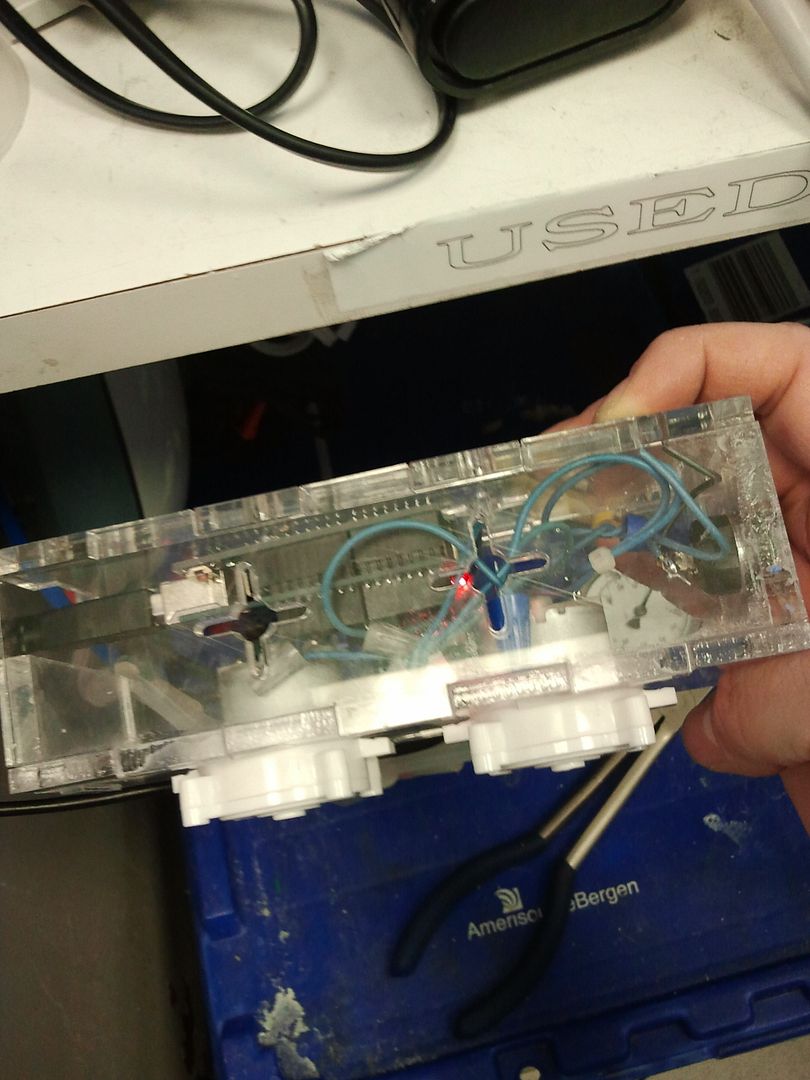
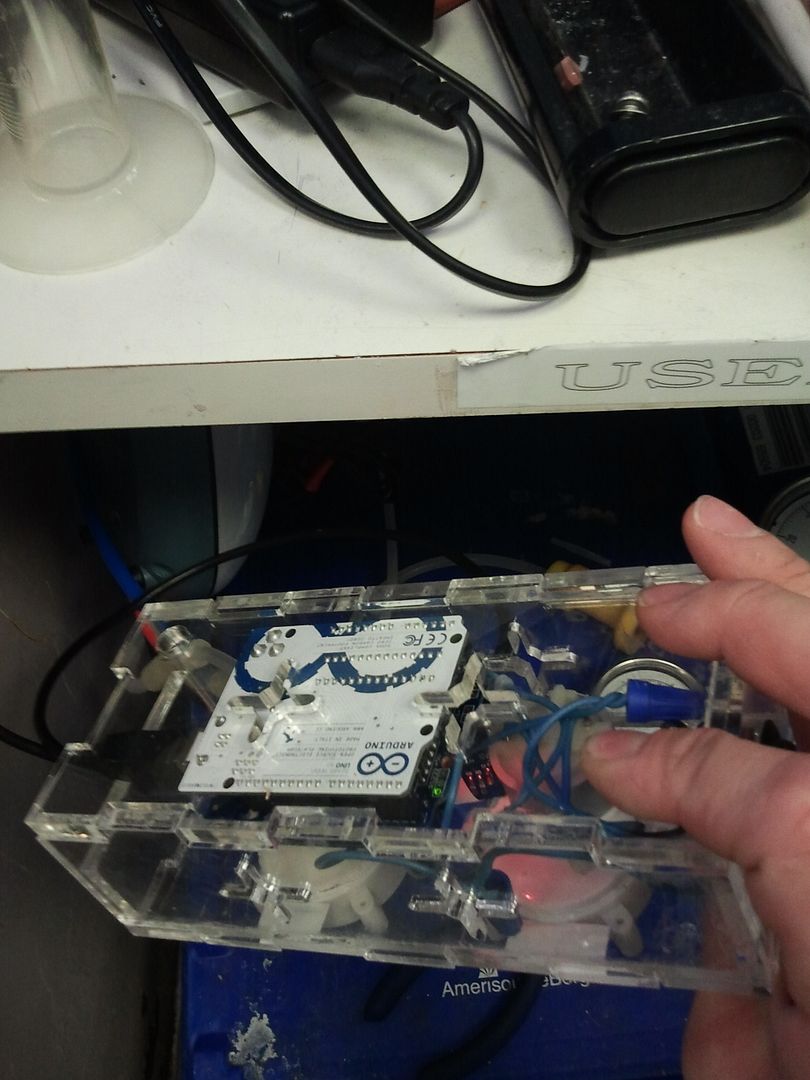
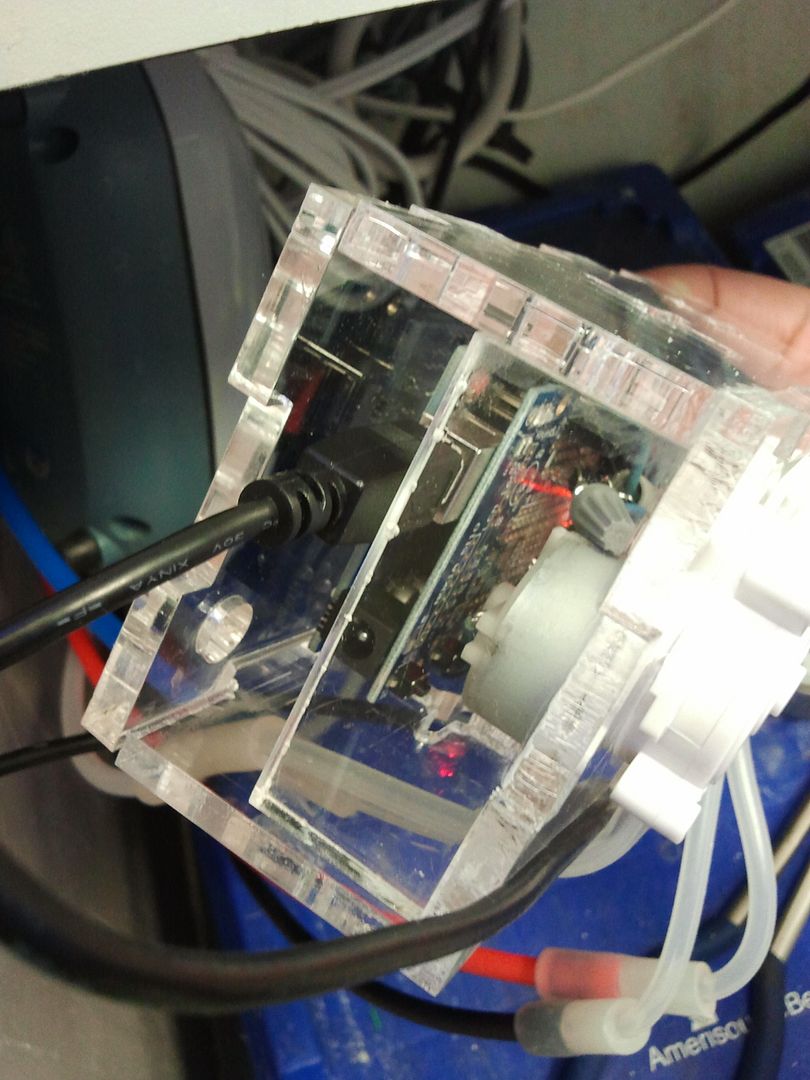
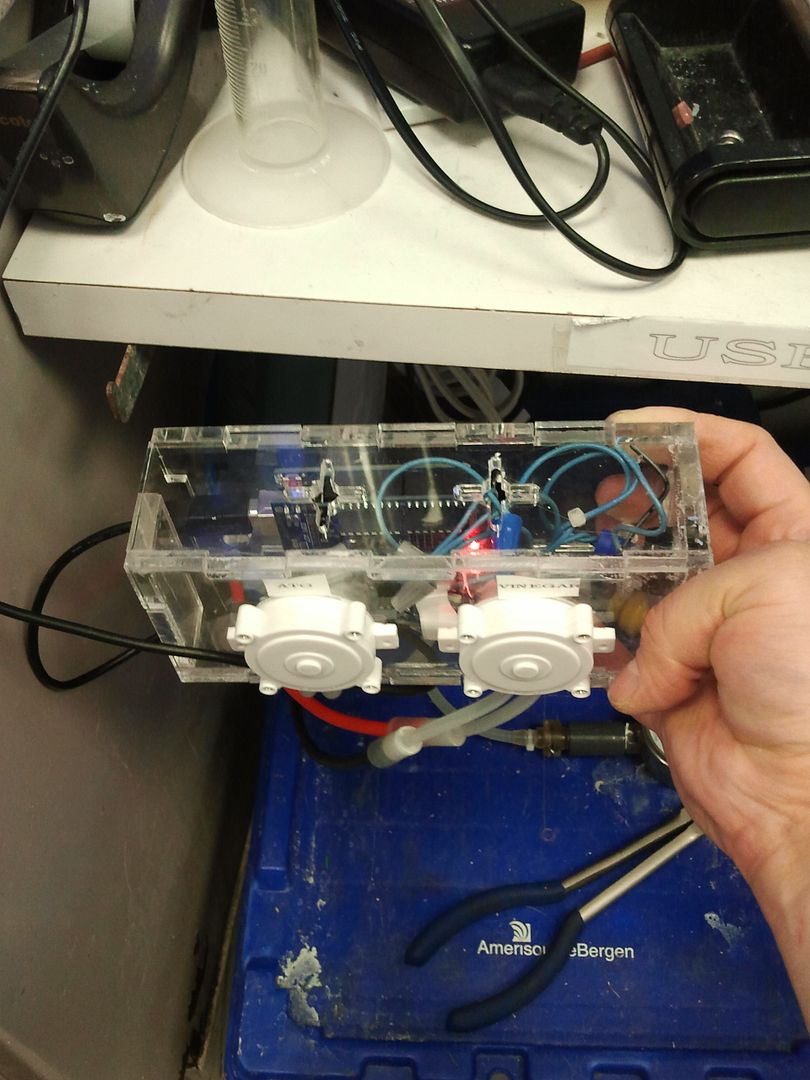
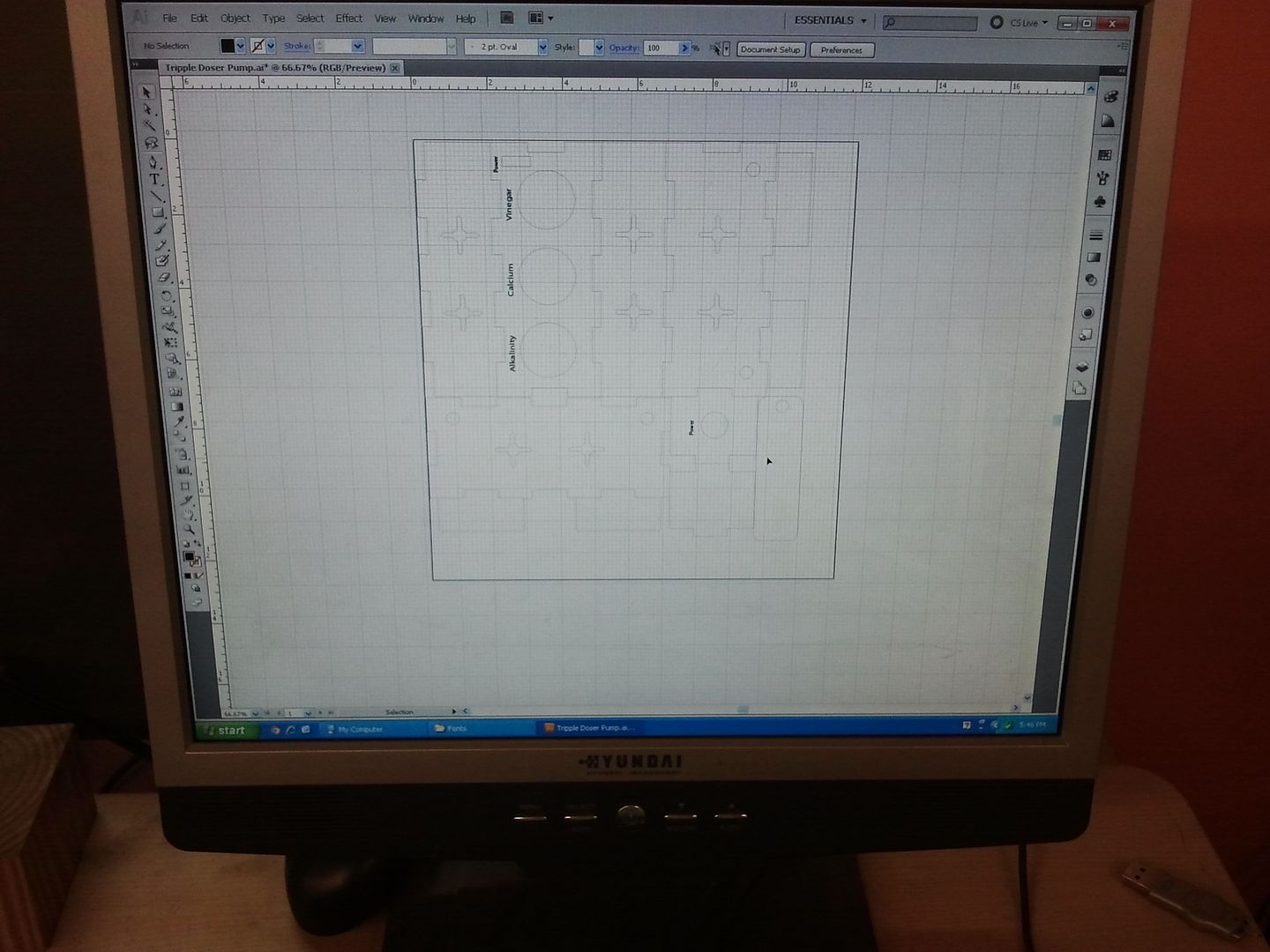
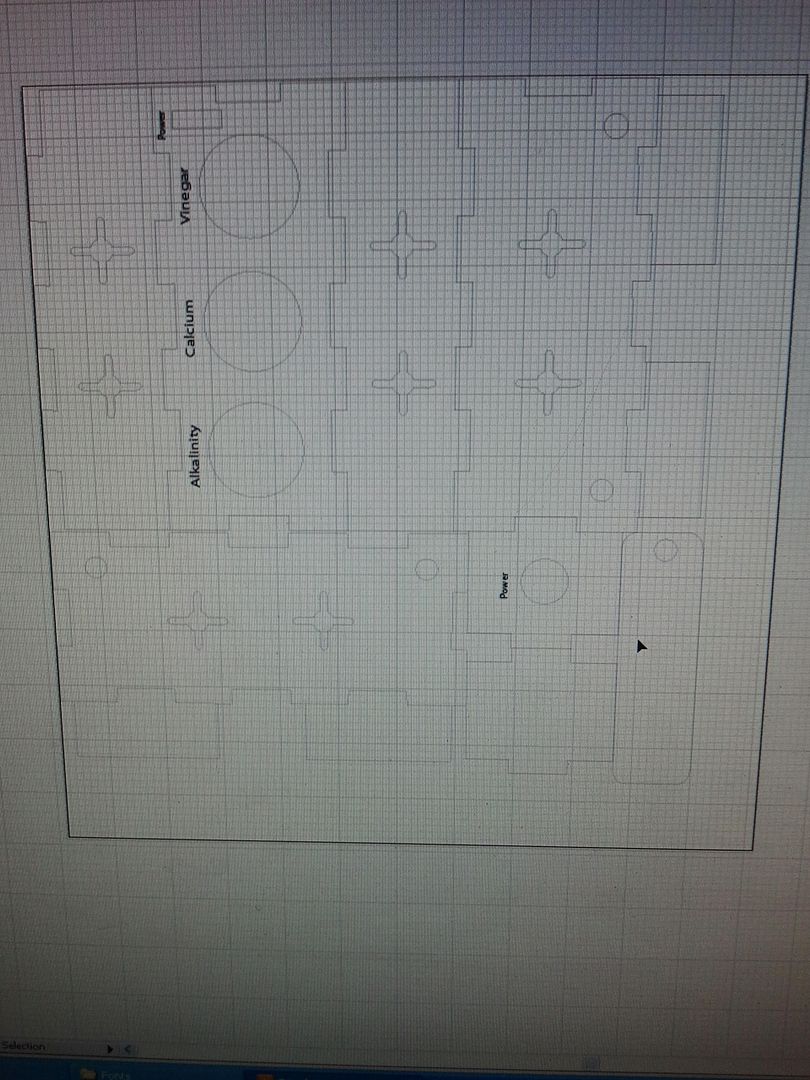

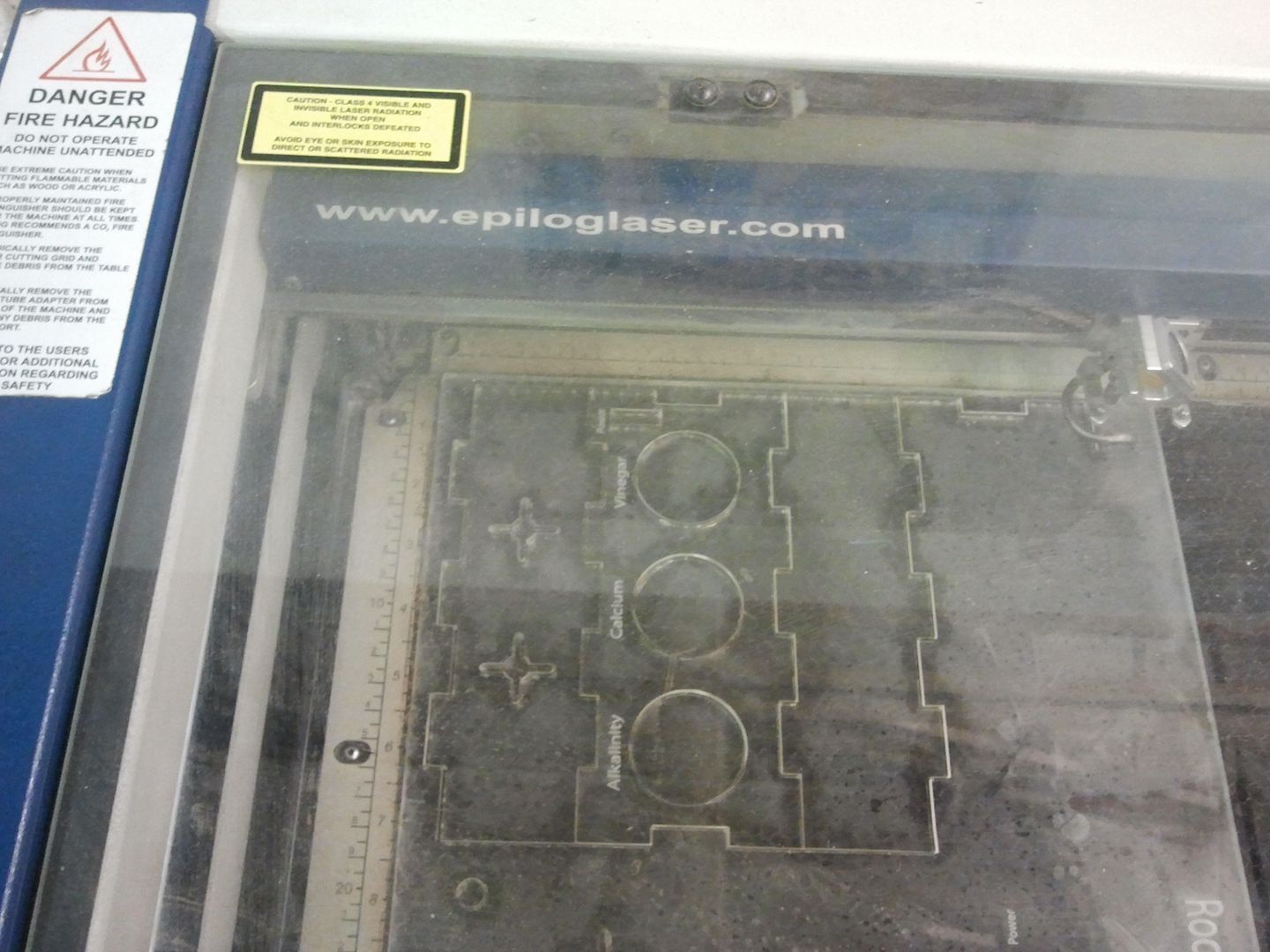
Files :
https://mega.co.nz/#!AZAHGBiT!FSgOTR1c_y0xpakP-_suoHZv09VBAQTTLZ390Gas-RY
I ordered these pumps:
http://www.ebay.com/itm/201020653246?ssPageName=STRK:MEWNX:IT&_trksid=p3984.m1497.l2649
25-30 ml a minute so its very slow dosing.
If anyone can improve on this please post suggestions and maybe we can make a better doser. Maybe with a LCD screen with buttons to change dosing and times. This code only allows you to program the dosing through the arduino coding program. The original site i got this from is not being updated, i tried to conact the owner for help on the code and i got no response. But thanks to him im learning how to use a Ardunio.